Appearance
文件上传
自定义接口支持任意binary格式和 form-data
格式的文件上传接口。 入参形式如下:
Blob
用于接收文件流,如图片,音频等。 接收参数固定为
$data
ts@action.param({ type: "Blob", required: true, description: "文件流" }) $data: any;
File
用于接收
multipart/form-data
格式数据的结构体, 当用 custom api 封装文件上传接口时可以使用。ts@action.param({ type: "File", required: true, description: "form data 数据" }) file: http.FormData;
上传 binary 文件, 请求体即文件的所有内容
新建脚本并激活
此脚本接收任意文件内容, 并将其上传到 minio 中, 最终返回在minio中的访问地址。
ts
import * as obs from 'objectstorage';
export class Input {
// 文件的所有数据将赋值到 $data 变量上
@action.param({ type: "Blob", required: true, description: "文件流" })
$data: any;
}
export class Output {
endpoint: string;
}
export class FileUploader {
@action.method({ input: "Input", output: "Output", description: "file upload" })
run(input: Input): Output {
console.log("input data length: ", input.$data.length);
let output = new Output();
let cli = obs.newClient(obs.StoreType.MINIO, "cyl__minio");
let name = "test1.png";
cli.uploadObject(name, input.$data, {});
console.log(cli.getEndpoint() + name);
output.endpoint = cli.getEndpoint() + name;
return output;
}
}
新建自定义接口并绑定上述脚本
注意内容类型为 binary-data
,方法固定为 POST
测试上传
可以用 vscode rest 插件来发送请求
POST https://{host}:{port}/service/cyl__LostXamd/1.0.0/upload/binary
Content-Type: image/png
Access-Token: {{token}}
< {local_file_path}
上传结果
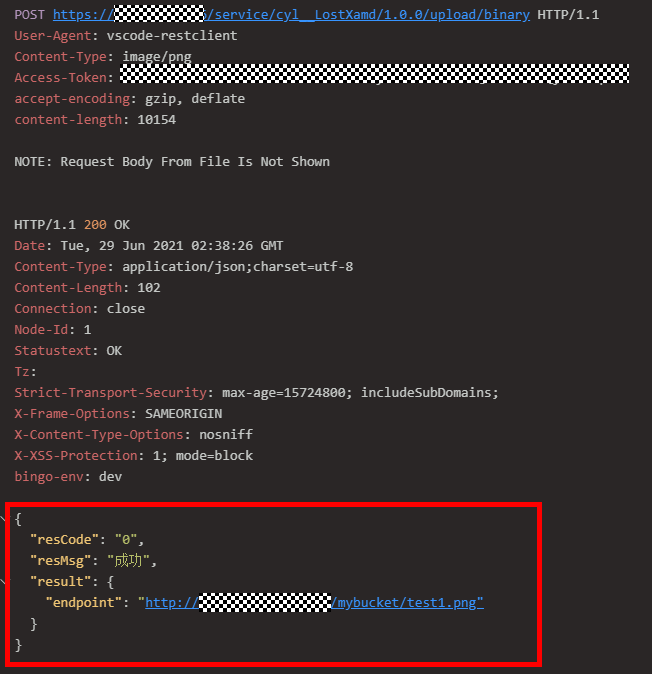
到minio上查看结果
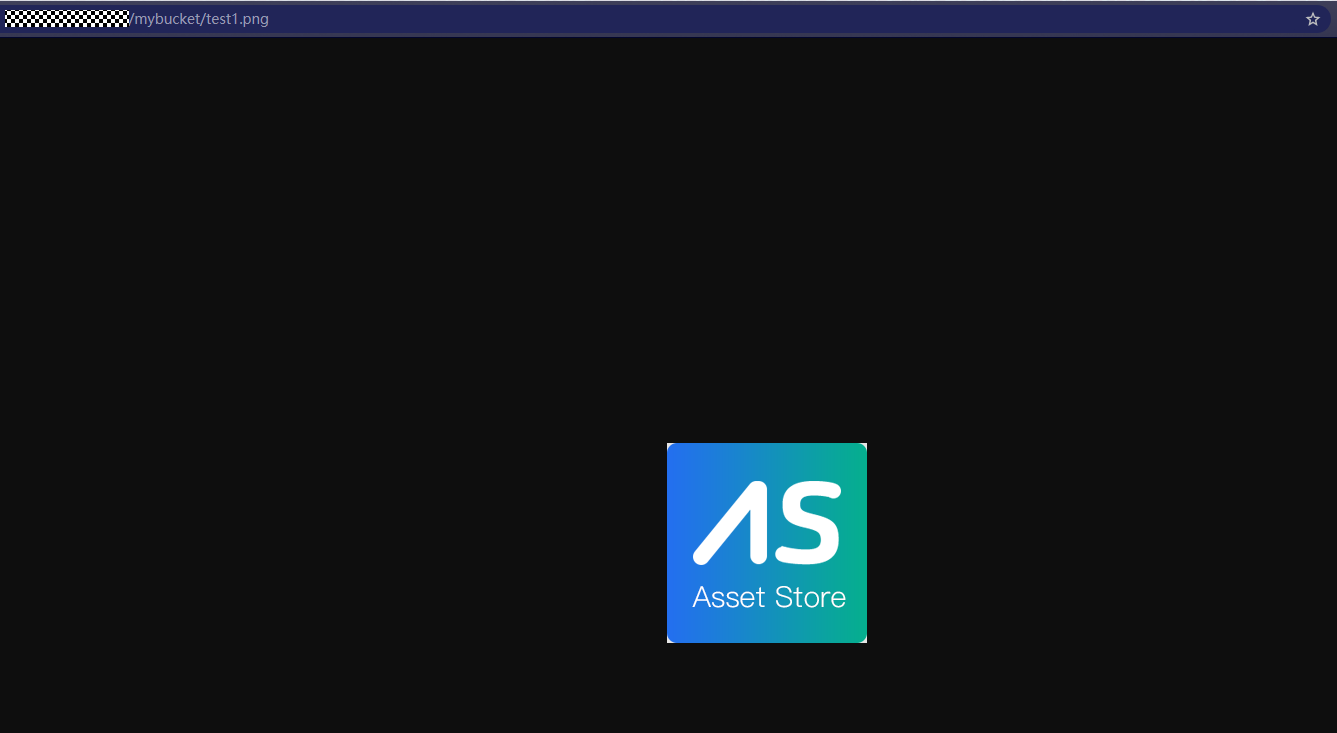
通过 Form-Data 格式上传文件
新建脚本并激活
此脚本接收 form-data
格式请求, 第一个参数 name 表示最终要保存的文件名; 第二个参数 file 是 http.FormData 类型的参数, 包含文件名,文件内容和文件类型
ts
import * as obs from 'objectstorage';
import * as http from 'http';
export class Input {
@action.param({ type: "String", required: true, description: "文件名" })
name: string;
@action.param({ type: "File", required: true, description: "form data 数据" })
file: http.FormData;
}
export class Output {
endpoint: string;
}
export class FileUploaderFormData {
@action.method({ input: "Input", output: "Output", description: "do a operation" })
run(input: Input): Output {
console.log("input data length: ", input.file.data.length);
let output = new Output();
let cli = obs.newClient(obs.StoreType.MINIO, "cyl__minio");
let name = input.name;
cli.uploadObject(name, input.file.data, {});
console.log(cli.getEndpoint() + name);
output.endpoint = cli.getEndpoint() + name;
return output;
}
}
创建自定义接口并绑定脚本
注意内容类型为 multipart/form-data
测试上传 form-data
###
POST https://{host}:{port}/service/cyl__LostXamd/1.0.0/upload/formdata
Access-Token: {{token}}
Content-Type: multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="file"; filename="1.png"
< {local_host_path}
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="name"
testformdata.png
------WebKitFormBoundary7MA4YWxkTrZu0gW--
上传结果